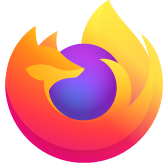
Javascript compatibility problem with disco background effect (works on IE but not FF)
This is the code:
r=255; g=255; b=255; flag=0; t=new Array; o=new Array; d=new Array;
function hex(a,c) { t[a]=Math.floor(c/16) o[a]=c%16 switch (t[a]) { case 10: t[a]='A'; break; case 11: t[a]='B'; break; case 12: t[a]='C'; break; case 13: t[a]='D'; break; case 14: t[a]='E'; break; case 15: t[a]='F'; break; default: break; } switch (o[a]) { case 10: o[a]='A'; break; case 11: o[a]='B'; break; case 12: o[a]='C'; break; case 13: o[a]='D'; break; case 14: o[a]='E'; break; case 15: o[a]='F'; break; default: break; } }
function ran(a,c) { if ((Math.random()>2/3||c==0)&&c<255) { c++ d[a]=2; } else { if ((Math.random()<=1/2||c==255)&&c>0) { c-- d[a]=1; } else d[a]=0; } return c } function do_it(a,c) { if ((d[a]==2&&c<255)||c==0) { c++ d[a]=2 } else if ((d[a]==1&&c>0)||c==255) { c--; d[a]=1; } if (a==3) { if (d[1]==0&&d[2]==0&&d[3]==0) flag=1 } return c } function disco() { if (flag==0) { r=ran(1, r); g=ran(2, g); b=ran(3, b); hex(1,r) hex(2,g) hex(3,b) document.body.style.background="#"+t[1]+o[1]+t[2]+o[2]+t[3]+o[3] flag=50 } else { r=do_it(1, r) g=do_it(2,g) b=do_it(3,b) hex(1,r) hex(2,g) hex(3,b) document.body.style.background="#"+t[1]+o[1]+t[2]+o[2]+t[3]+o[3] flag-- }
setTimeout('disco()',50) }
Can someone please tell me how to make this work in Firefox also, because I'm designing a website for which I'd love to put this as a background, but if I'm to do it, it needs to be compatible with all the major browsers.
Chosen solution
Seems to be working here. Be careful using % in c%16 -> c % 16. Otherwise %16 might get unescaped.
data:text/html;charset=utf-8, <html> <head> <script> var r=255,g=255,b=255,flag=0,t=new Array,o=new Array,d=new Array; function hex(a,c) { t[a]=Math.floor(c/16);o[a]=c % 16; switch (t[a]) { case 10:t[a]='A';break; case 11:t[a]='B';break; case 12:t[a]='C';break; case 13:t[a]='D';break; case 14:t[a]='E';break; case 15:t[a]='F';break; default:break; } switch (o[a]) { case 10:o[a]='A';break; case 11:o[a]='B';break; case 12:o[a]='C';break; case 13:o[a]='D';break; case 14:o[a]='E';break; case 15:o[a]='F';break; default:break; } } function ran(a,c) { if ((Math.random()> 2/3 || c==0) && c<255){c++;d[a]=2;} else{if ((Math.random() <= 1/2 || c==255) && c>0){c--;d[a]=1;}else d[a]=0;} return c } function do_it(a,c) { if ((d[a]==2 && c<255)|| c==0){c++;d[a]=2;} else if ((d[a]==1 && c>0) || c==255){c--;d[a]=1;} if (a==3) {if (d[1]==0 && d[2]==0 && d[3]==0)flag=1;} return c } function disco() { if (flag==0) { r=ran(1, r);g=ran(2, g);b=ran(3, b); hex(1,r);hex(2,g);hex(3,b); document.body.style.background="#"+t[1]+o[1]+t[2]+o[2]+t[3]+o[3]; flag=50; } else { r=do_it(1, r);g=do_it(2,g);b=do_it(3,b); hex(1,r);hex(2,g);hex(3,b); document.body.style.background="#"+t[1]+o[1]+t[2]+o[2]+t[3]+o[3]; flag--; } setTimeout('disco()',50); } </script> </head> <body style="height:100%;" onload="disco();"> </body> </html>Read this answer in context 👍 1
All Replies (5)
Chosen Solution
Seems to be working here. Be careful using % in c%16 -> c % 16. Otherwise %16 might get unescaped.
data:text/html;charset=utf-8, <html> <head> <script> var r=255,g=255,b=255,flag=0,t=new Array,o=new Array,d=new Array; function hex(a,c) { t[a]=Math.floor(c/16);o[a]=c % 16; switch (t[a]) { case 10:t[a]='A';break; case 11:t[a]='B';break; case 12:t[a]='C';break; case 13:t[a]='D';break; case 14:t[a]='E';break; case 15:t[a]='F';break; default:break; } switch (o[a]) { case 10:o[a]='A';break; case 11:o[a]='B';break; case 12:o[a]='C';break; case 13:o[a]='D';break; case 14:o[a]='E';break; case 15:o[a]='F';break; default:break; } } function ran(a,c) { if ((Math.random()> 2/3 || c==0) && c<255){c++;d[a]=2;} else{if ((Math.random() <= 1/2 || c==255) && c>0){c--;d[a]=1;}else d[a]=0;} return c } function do_it(a,c) { if ((d[a]==2 && c<255)|| c==0){c++;d[a]=2;} else if ((d[a]==1 && c>0) || c==255){c--;d[a]=1;} if (a==3) {if (d[1]==0 && d[2]==0 && d[3]==0)flag=1;} return c } function disco() { if (flag==0) { r=ran(1, r);g=ran(2, g);b=ran(3, b); hex(1,r);hex(2,g);hex(3,b); document.body.style.background="#"+t[1]+o[1]+t[2]+o[2]+t[3]+o[3]; flag=50; } else { r=do_it(1, r);g=do_it(2,g);b=do_it(3,b); hex(1,r);hex(2,g);hex(3,b); document.body.style.background="#"+t[1]+o[1]+t[2]+o[2]+t[3]+o[3]; flag--; } setTimeout('disco()',50); } </script> </head> <body style="height:100%;" onload="disco();"> </body> </html>
Modified
Wow! Thanks a lot. Now it works in FF :-)
But only downside is it no longer works in IE. We all know IE doesn't follow W3C standards (transparency is such a pain in IE because it doesn't use rbga colors) and it's pretty much off its rocker, but this is just crazy.
Can you please, by any chance make your code run for both IE and FF or do I need to detect browser and run one of the two scripts according to the user's browser?
I'm on Linux, so can't test it in IE. I would assume that only document.body.style.background could cause problems in IE. The rest is pretty standard. I only added some spaces and semicolons to make your code work as a data URI to test it.
A good place to ask questions and advice about web development is at the mozillaZine Web Development/Standards Evangelism forum.
The helpers at that forum are more knowledgeable about web development issues.
You need to register at the mozillaZine forum site in order to post at that forum.
See http://forums.mozillazine.org/viewforum.php?f=25
Thanks a lot for your great help :-) I just used JavaScript to run your corrected script for FF users and the old one that ran on IE for IE users and so it's functioning just fine. I will look into the forums as you said for a more technical response regarding this. Thanks a million.
You're welcome